class anychart.graphics.vector.Layer Improve this Doc
Extends: anychart.graphics.vector.Element
Layer class. Used to group elements.
Elements must be grouped if you want to apply similar changes to them,
for example transformation.
Do not invoke constructor directly. Use anychart.graphics.vector.Stage#layer
to create stage bound layer.
If you want to create an unbound
layer - use anychart.graphics#layer
See also:
anychart.graphics.vector.Stage#layer,
anychart.graphics#layer
Elements indices (and layers indices within a stage) set Z-order.
The "higher" an element is, the greater its index.
Try it:
Methods Overview
Events | |
listen() | Adds an event listener. |
listenOnce() | Adds an event listener that is removed automatically after the listener fired once. |
removeAllListeners() | Removes all listeners from this listenable. |
unlisten() | Removes an event listener which was added with listen() or listenOnce(). |
Interactive | |
drag() | Dragging setting. |
Interactivity | |
visible() | Visibility flag |
Size and Position | |
appendTransformationMatrix() | Combines the transformation with the given transformation matrix. |
getAbsoluteBounds() | Gets element bounds in absolute coordinates (root element coordinate system). |
getAbsoluteHeight() | Returns height within root bounds. |
getAbsoluteWidth() | Returns width within root bounds. |
getAbsoluteX() | Returns an absolute X (root element coordinate system). |
getAbsoluteY() | Returns an absolute Y (root element coordinate system). |
getBounds() | Returns the bounds. |
getHeight() | Returns the height. |
getRotationAngle() | Returns the rotation angle in degrees. |
getTransformationMatrix() | Returns the transformation matrix. |
getWidth() | Returns the width. |
getX() | Returns X in the coordinate system of the parent. |
getY() | Returns Y in the coordinate system of the parent. |
rotate() | Rotates a shape around the given rotation point. |
rotateByAnchor() | Rotates a shape around the given anchor. |
scale() | Scales a shape. Scaling center is set in the coordinate system of the parent. |
scaleByAnchor() | Scales a shape by anchor. Scaling center is set as an anchor. |
setPosition() | Sets top left corner of a shape (transformation taken into account) in the coordinate system of the parent. |
setRotation() | Rotates a shape around the given point. |
setRotationByAnchor() | Rotates a shape around the given anchor. |
setTransformationMatrix() | Sets the transformation matrix. |
translate() | Moves a shape taking an account the transformation. |
zIndex() | Z-index setting. |
Miscellaneous | |
addChild() | Add element to a layer, to the top (maximal index). |
addChildAt() | Adds an element to a layer by index. |
attr() | Attribute setting |
circle() | Invokes anychart.graphics.vector.Circle class constructor. |
clip() | Clipping setting. |
cross() | Draws a cross set by its circumscribed circle center and radius. |
cursor() | Cursor type. |
desc() | Description setting |
diagonalCross() | Draws a diagonal cross set by its circumscribed circle center and radius. |
diamond() | Draws a diamond set by its circumscribed circle center and radius. |
disablePointerEvents() | Pointer events setting. |
disableStrokeScaling() | Vector effect property |
dispose() | Disposes element completely. |
domElement() | Returns DOM element if element is rendered. |
donut() | Draws sector as donut chart element. |
ellipse() | Invokes anychart.graphics.vector.Ellipse class constructor. |
forEachChild() | Applies function to all elements in a layer. |
getChildAt() | Returns element by index. |
getStage() | Stage object (to which the given element is bound). |
hLine() | Draws a thick horizontal line set by its circumscribed circle center and radius. |
hasChild() | Checks if there is such element in children set. |
hasParent() | Whether parent element is set. |
html() | Invokes anychart.graphics.vector.Text class constructor and applies anychart.graphics.vector.Text#htmlText method |
id() | Element identifier |
image() | Invokes anychart.graphics.vector.Image class constructor. |
indexOfChild() | Looks for an element in a layer and returns index or -1, if not found. |
layer() | Invokes anychart.graphics.vector.Layer class constructor. |
numChildren() | Returns the number of children. |
parent() | Parent layer. |
path() | Invokes anychart.graphics.vector.Path class constructor. |
pie() | Draws sector as pie chart element. |
rect() | Invokes anychart.graphics.vector.Rect class constructor. |
remove() | Current element removes itself from the parent layer. |
removeChild() | Removes element from a layer. |
removeChildAt() | Removes element from a layer by index. |
removeChildren() | Removes all children from a layer. |
roundedInnerRect() | Draws rectangle with corners rounded inside. |
roundedRect() | Draws rectangle with rounded corners. |
star() | Draws multi-pointed star. |
star10() | Draws ten-pointed star. |
star4() | Draws four-pointed star. |
star5() | Draws five-pointed star. |
star6() | Draws six-pointed star. |
star7() | Draws seven-pointed star. |
swapChildren() | Swaps children. |
swapChildrenAt() | Swaps children by indexes. |
text() | Invokes anychart.graphics.vector.Text class constructor. |
title() | Title setting |
triangleDown() | Draws a triangle heading downwards set by its circumscribed circle center and radius. |
triangleLeft() | Draws a triangle heading leftwards set by its circumscribed circle center and radius. |
triangleRight() | Draws a triangle heading rightwards set by its circumscribed circle center and radius. |
triangleUp() | Draws a triangle heading upwards set by its circumscribed circle center and radius. |
truncatedRect() | Draws rectangle with cut corners. |
vLine() | Draws a thick vertical line set by its circumscribed circle center and radius. |
Methods Description
addChild
All DOM changes will happen instantly, except anychart.graphics.vector.Stage#suspend.
Simplified version of anychart.graphics.vector.Layer#addChildAt where element is added to the end.
Params:
Name | Type | Description |
---|---|---|
element | anychart.graphics.vector.Element | Element to add. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
addChildAt
Note: the greater index is - the 'higher' element is in a layer top element overlaps bottom elements.
All DOM changes will happen instantly, except anychart.graphics.vector.Stage#suspend.
Left image shows sequential calls of anychart.graphics.vector.Layer#addChild
Right image does the same, but star is added to 0 index.
.addChildAt(star5, 0);
(see code of this image in samples).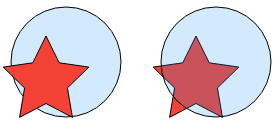
Params:
Name | Type | Description |
---|---|---|
element | anychart.graphics.vector.Element | Element to add. |
index | number | Element index. Only positive numbers. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
appendTransformationMatrix
Illustration shows a shape transformation with the several calls of
.appendTransformationMatrix(0, 0.5, 1, 0, 0, 0)
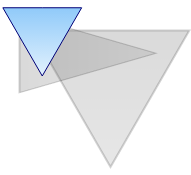
Params:
Name | Type | Description |
---|---|---|
m00 | number | Scale X. |
m10 | number | Shear Y. |
m01 | number | Shear X. |
m11 | number | Scale Y. |
m02 | number | Translate X. |
m12 | number | Translate Y. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
attr
Params:
Name | Type | Description |
---|---|---|
key | string | Name of attribute. |
Returns:
* - Attribute value.Try it:
Params:
Name | Type | Description |
---|---|---|
key | string | Name of attribute. |
value | * | Value of attribute. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
circle
Note:
anychart.graphics.vector.Layer does nothing to delete an object after it is used. You have to take care of used objects yourself.
Read more at anychart.graphics.vector.Circle
Params:
Name | Type | Description |
---|---|---|
cx | number | Center X, in pixels. |
cy | number | Center Y, in pixels. |
radius | number | Radius, in pixels. |
Returns:
anychart.graphics.vector.Circle - An instance of the class for method chaining.Try it:
clip
Returns:
anychart.graphics.math.Rect - Returns rectangle settings.Attention! In SVG clip will transform according to transformation, and in VML clip will be surrounding.
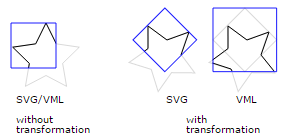
Params:
Name | Type | Description |
---|---|---|
value | anychart.graphics.math.Rect | string | Clipping rectangle. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
cross
Read more at anychart.graphics.vector.primitives#cross
Try it:
cursor
Params:
Name | Type | Default | Description |
---|---|---|---|
value | anychart.graphics.vector.Cursor | null | Cursor type. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
desc
Returns:
string | null | undefined - The element description value.Try it:
Params:
Name | Type | Description |
---|---|---|
value | string | null | Value to set. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
diagonalCross
Read more at anychart.graphics.vector.primitives#diagonalCross
Try it:
diamond
Read more at anychart.graphics.vector.primitives#diamond
Try it:
disablePointerEvents
Params:
Name | Type | Default | Description |
---|---|---|---|
value | boolean | false | Pointer events property value. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
disableStrokeScaling
Returns:
boolean - The vector effect property.var element = stage.rect(10, 10, 100, 40); var disableStrokeScaling = element.disableStrokeScaling();
Learn more by link.
Params:
Name | Type | Default | Description |
---|---|---|---|
enabled | boolean | false | Vector effect property to set. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
dispose
Try it:
domElement
In case of Stage in Suspended state or unbound element - null is returned.
Returns:
Element - DOM element.donut
Read more at anychart.graphics.vector.primitives#donut
Try it:
drag
Returns:
boolean | anychart.graphics.math.Rect - Absolute element bounds or flag.Try it:
Params:
Name | Type | Default | Description |
---|---|---|---|
value | boolean | anychart.graphics.math.Rect | false | Flag or a dragging area. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
ellipse
Note:
anychart.graphics.vector.Layer does nothing to delete an object after it is used. You have to take care of used objects yourself.
Read more at anychart.graphics.vector.Ellipse
Params:
Name | Type | Description |
---|---|---|
cx | number | Center X, in pixels. |
cy | number | Center Y, in pixels. |
rx | number | Radius X, in pixels. |
ry | number | Radius Y, in pixels. |
Returns:
anychart.graphics.vector.Ellipse - An instance of the class for method chaining.Try it:
forEachChild
Params:
Name | Type | Description |
---|---|---|
callback | function(element:anychart.graphics.vector.Element):void | Function to be applied. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
Params:
Name | Type | Description |
---|---|---|
callback | function(element:anychart.graphics.vector.Element):void | Function to be applied. |
this | Object | this context for the function to be applied. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.getAbsoluteBounds
Returns:
anychart.graphics.math.Rect - Absolute element bounds.Try it:
getAbsoluteHeight
See illustrations at anychart.graphics.vector.Element#getAbsoluteWidth
Returns:
number - Height.Try it:
getAbsoluteWidth
getAbsoluteX
Returns:
number - Absolute X.Try it:
getAbsoluteY
Returns:
number - Absolute Y.Try it:
getBounds
getChildAt
Params:
Name | Type | Description |
---|---|---|
index | number | Element to be returned. |
Returns:
anychart.graphics.vector.Layer - Element or null.Try it:
getHeight
getRotationAngle
getStage
Returns:
anychart.graphics.vector.Stage - Stage object.Try it:
getTransformationMatrix
[
{number} m00 Scale X.
{number} m10 Shear Y.
{number} m01 Shear X.
{number} m11 Scale Y.
{number} m02 Translate X.
{number} m12 Translate Y.
]
Returns:
Array.<number> - Transformation matrix array.Try it:
getWidth
getX
Returns:
number - X in the coordinate system of the parent.Try it:
getY
Returns:
number - Y in the coordinate system of the parent.Try it:
hLine
Read more at anychart.graphics.vector.primitives#hLine
Try it:
hasChild
Params:
Name | Type | Description |
---|---|---|
element | anychart.graphics.vector.Element | Element. |
Returns:
boolean - True if it is a child.hasParent
html
Note:
anychart.graphics.vector.Layer does nothing to delete an object after it is used. You have to take care of used objects yourself.
Params:
Name | Type | Description |
---|---|---|
x | number | X-coordinate (Left) of top left corner of text bounds. |
y | number | Y-coordinate (Top) of top left corner of text bounds. |
text | string | Text to be displayed. |
style | anychart.graphics.vector.TextStyle | Text style. More at anychart.graphics.vector.Text#style. |
Returns:
anychart.graphics.vector.Text - An instance of the class for method chaining.Try it:
id
Returns:
string - Returns element identifier.Try it:
Params:
Name | Type | Description |
---|---|---|
id | string | Custom identifier. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
image
Note:
anychart.graphics.vector.Layer does nothing to delete an object after it is used. You need to take care of used objects yourself.
Params:
Name | Type | Description |
---|---|---|
src | string | IRI (Internationalized Resource Identifiers) for image source. |
x | number | X coordinate of left-top corner image. |
y | number | Y coordinate of left-top corner image. |
width | number | Width of image bounds. |
height | number | Height of image bounds. |
Returns:
anychart.graphics.vector.Image - Image object instance.Try it:
indexOfChild
Params:
Name | Type | Description |
---|---|---|
element | anychart.graphics.vector.Element | Element which index we need to find. |
Returns:
number - Index or -1, or not found.Try it:
layer
Note:
anychart.graphics.vector.Layer does nothing to delete an object after it is used. You have to take care of used objects yourself.
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
listen
Params:
Name | Type | Default | Description |
---|---|---|---|
type | string | The event type id. | |
listener | function(e:Object):boolean|undefined | Callback method. | |
useCapture | boolean | false | Whether to fire in capture phase. Learn more about capturing https://javascript.info/bubbling-and-capturing |
listenerScope | Object | Object in whose scope to call the listener. |
Returns:
Object - Unique key for the listener.Try it:
listenOnce
Params:
Name | Type | Default | Description |
---|---|---|---|
type | string | The event type id. | |
listener | function(e:Object):boolean|undefined | Callback method. | |
useCapture | boolean | false | Whether to fire in capture phase. Learn more about capturing https://javascript.info/bubbling-and-capturing |
listenerScope | Object | Object in whose scope to call the listener. |
Returns:
Object - Unique key for the listener.Try it:
numChildren
parent
Returns:
anychart.graphics.vector.Layer | anychart.graphics.vector.Stage - Instance of element current layer.Try it:
Params:
Name | Type | Description |
---|---|---|
parent | anychart.graphics.vector.Layer | anychart.graphics.vector.Stage | Parent layer. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
path
Note:
anychart.graphics.vector.Layer does nothing to delete an object after it is used. You have to take care of used objects yourself.
Read more at: anychart.graphics.vector.Path
Returns:
anychart.graphics.vector.Path - An instance of the class for method chaining.Try it:
pie
Read more at anychart.graphics.vector.primitives#pie
Try it:
rect
Note:
anychart.graphics.vector.Layer does nothing to delete an object after it is used. You have to take care of used objects yourself.
Params:
Name | Type | Description |
---|---|---|
x | number | X (Left) of top left rectangle corner. |
y | number | Y (Top) of to left rectangle corner. |
width | number | Rectangle width. |
height | number | Rectangle height. |
Returns:
anychart.graphics.vector.Rect - An instance of the class for method chaining.Try it:
remove
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
removeAllListeners
Params:
Name | Type | Description |
---|---|---|
type | string | Type of event to remove, default is to remove all types. |
Returns:
number - Number of listeners removed.Try it:
removeChild
All changes in DOM will happen instantly, except anychart.graphics.vector.Stage#suspend.
Note: this method doesn't remove element, it just breaks the link between the element and the layer.
This is an extension of anychart.graphics.vector.Layer#removeChildAt method.
Params:
Name | Type | Description |
---|---|---|
element | anychart.graphics.vector.Element | Element to remove or its id. |
Returns:
anychart.graphics.vector.Layer - Removed element or null.Try it:
removeChildAt
All changes in DOM will happen instantly, except anychart.graphics.vector.Stage#suspend.
Note: this method doesn't remove element, it just breaks the link between the element and the layer.
Params:
Name | Type | Description |
---|---|---|
index | number | Index of element to be removed. |
Returns:
anychart.graphics.vector.Layer - Removed element or null.Try it:
removeChildren
Returns:
Array.<anychart.graphics.vector.Element> - Array of removed elements.Try it:
rotate
Left illustration shows 20 degrees rotation with the default rotation point.
.rotate(20)
Right illustration shows 20 degrees rotation with the given rotation point.
.rotate(20, x, y)
Rotation points are marked with red, initial position of shapes is marked with gray.
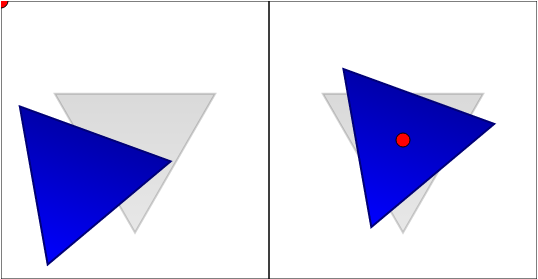
Params:
Name | Type | Description |
---|---|---|
degrees | number | Rotation angle in degrees. |
cx | number | Rotation point X. |
cy | number | Rotation point Y. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
rotateByAnchor
Left illustration shows 90 degrees rotation around the default anchor.
.rotateByAnchor(90)
Right illustration shows 90 degrees rotation around the given anchor.
.rotateByAnchor(90, anychart.graphics.vector.Anchor.CENTER_TOP)
Rotation points are marked with red, initial position of shapes is marked with gray.
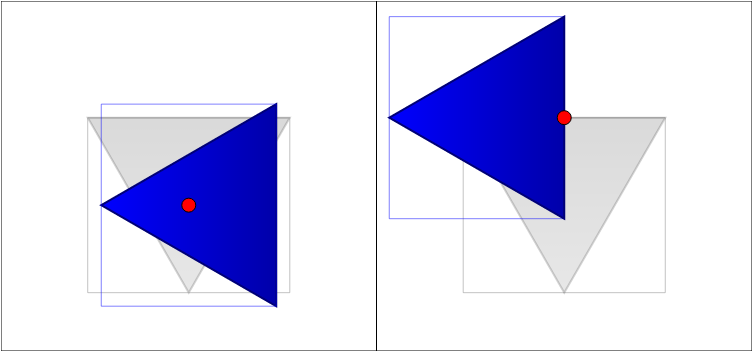
Params:
Name | Type | Description |
---|---|---|
degrees | number | Rotation angle in degrees. |
anchor | anychart.graphics.vector.Anchor | string | Rotation anchor. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
roundedInnerRect
Read more at anychart.graphics.vector.primitives#roundedInnerRect
Try it:
roundedRect
Read more at anychart.graphics.vector.primitives#roundedRect
Try it:
scale
Left illustration shows default point scaling.
.scale(0.5, 0.5,)
Right illustration shows scaling with the scaling center set.
.scale(0.5, 0.5, x, y)
Scaling center is marked with red, initial state is marked with gray.
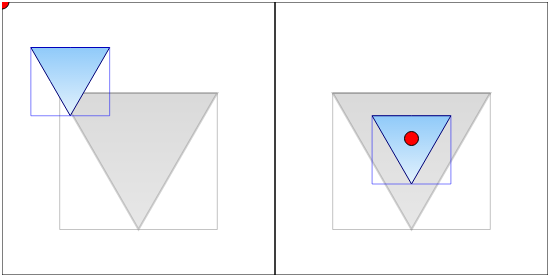
Params:
Name | Type | Description |
---|---|---|
sx | number | X scaling factor. |
sy | number | Y scaling factor. |
cx | number | Scaling point X. |
cy | number | Scaling point Y. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
scaleByAnchor
Left illustration shows scaling with the default anchor.
.scaleByAnchor(0.5, 0.5)
Right illustration shows scaling with the anchor set.
.scaleByAnchor(0.5, 0.5, anychart.graphics.vector.Anchor.RIGHT_TOP)
Scaling center is marked with red, initial state is marked with gray.
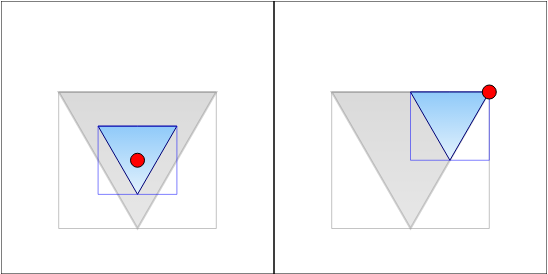
Params:
Name | Type | Description |
---|---|---|
sx | number | X scaling factor. |
sy | number | Y scaling factor. |
anchor | anychart.graphics.vector.Anchor | string | Scaling anchor point. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
setPosition
Params:
Name | Type | Description |
---|---|---|
x | number | X coordinate. |
y | number | Y coordinate. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
setRotation
Params:
Name | Type | Description |
---|---|---|
degrees | number | Rotation angle in degrees. |
cx | number | Rotation point X. |
cy | number | Rotation point Y. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
setRotationByAnchor
Params:
Name | Type | Description |
---|---|---|
degrees | number | Rotation angle in degrees. |
anchor | anychart.graphics.vector.Anchor | string | Rotation anchor. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
setTransformationMatrix
Params:
Name | Type | Description |
---|---|---|
m00 | number | Scale X. |
m10 | number | Shear Y. |
m01 | number | Shear X. |
m11 | number | Scale Y. |
m02 | number | Translate X. |
m12 | number | Translate Y. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
star
Read more at anychart.graphics.vector.primitives#star
Try it:
star10
Read more at anychart.graphics.vector.primitives#star10
Try it:
star4
Read more at anychart.graphics.vector.primitives#star4
Try it:
star5
Read more at anychart.graphics.vector.primitives#star5
Try it:
star6
Read more at anychart.graphics.vector.primitives#star6
Try it:
star7
Read more at anychart.graphics.vector.primitives#star7
Try it:
swapChildren
Params:
Name | Type | Description |
---|---|---|
element1 | anychart.graphics.vector.Element | First element. |
element2 | anychart.graphics.vector.Element | Second element. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
swapChildrenAt
Params:
Name | Type | Description |
---|---|---|
index1 | number | First element index. |
index2 | number | Second element index. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
text
Note:
anychart.graphics.vector.Layer does nothing to delete an object after it is used. You have to take care of used objects yourself.
Params:
Name | Type | Description |
---|---|---|
x | number | X-coordinate (Left) of left top corner of text bounds. |
y | number | Y-coordinate (Top) of left top corner of text bounds. |
text | string | Text to be displayed. |
style | anychart.graphics.vector.TextStyle | Text style. More at anychart.graphics.vector.Text#style. |
Returns:
anychart.graphics.vector.Text - An instance of the class for method chaining.title
Returns:
string | null | undefined - The element title value.Try it:
Params:
Name | Type | Description |
---|---|---|
value | string | null | Value to set. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
translate
Illustration shows a shape movement when
.translate(20, 10)
is invoked several times.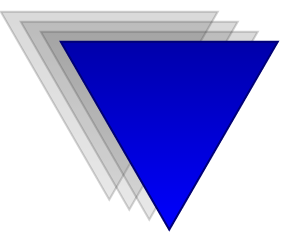
Params:
Name | Type | Description |
---|---|---|
tx | number | X movement amount. |
ty | number | Y movement amount. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
triangleDown
Read more at anychart.graphics.vector.primitives#triangleDown
Try it:
triangleLeft
Read more at anychart.graphics.vector.primitives#triangleLeft
Try it:
triangleRight
Read more at anychart.graphics.vector.primitives#triangleRight
Try it:
triangleUp
Read more at anychart.graphics.vector.primitives#triangleUp
Try it:
truncatedRect
Read more at anychart.graphics.vector.primitives#truncatedRect
Try it:
unlisten
Params:
Name | Type | Default | Description |
---|---|---|---|
type | string | The event type id. | |
listener | function(e:Object):boolean|undefined | Callback method. | |
useCapture | boolean | false | Whether to fire in capture phase. Learn more about capturing https://javascript.info/bubbling-and-capturing |
listenerScope | Object | Object in whose scope to call the listener. |
Returns:
boolean - Whether any listener was removed.Try it:
vLine
Read more at anychart.graphics.vector.primitives#vLine
Try it:
visible
Params:
Name | Type | Default | Description |
---|---|---|---|
isVisible | boolean | true | Visibility flag. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it:
zIndex
Params:
Name | Type | Description |
---|---|---|
value | number | Z-index to set. |
Returns:
anychart.graphics.vector.Layer - Self instance for method chaining.Try it: