class anychart.graphics.vector.Shape Improve this Doc
Extends: anychart.graphics.vector.Element
Base class for all vector elements.
Never invoke constructor directly!
Methods Overview
Coloring | |
fill() | Fill settings. |
stroke() | Stroke settings. |
Events | |
listen() | Adds an event listener. |
listenOnce() | Adds an event listener that is removed automatically after the listener fired once. |
removeAllListeners() | Removes all listeners from this listenable. |
unlisten() | Removes an event listener which was added with listen() or listenOnce(). |
Interactive | |
drag() | Dragging setting. |
Interactivity | |
visible() | Visibility flag |
Size and Position | |
appendTransformationMatrix() | Combines the current transformation with the given transformation matrix. |
getAbsoluteBounds() | Gets element bounds in absolute coordinates (root element coordinate system). |
getAbsoluteHeight() | Returns height within root bounds. |
getAbsoluteWidth() | Returns width within root bounds. |
getAbsoluteX() | Returns an absolute X (root element coordinate system). |
getAbsoluteY() | Returns an absolute Y (root element coordinate system). |
getBounds() | Returns the bounds. |
getHeight() | Returns the height. |
getRotationAngle() | Returns the current rotation angle in degrees. |
getTransformationMatrix() | Returns the current transformation matrix. |
getWidth() | Returns the width. |
getX() | Returns X in the coordinate system of the parent. |
getY() | Returns Y in the coordinate system of the parent. |
rotate() | Rotates a shape around the given rotation point. |
rotateByAnchor() | Rotates a shape around the given anchor. |
scale() | Scales a shape. Scaling center is set in the coordinate system of the parent. |
scaleByAnchor() | Scales a shape by anchor. Scaling center is set as an anchor. |
setPosition() | Sets top left corner of a shape (transformation taken into account) in the coordinate system of the parent. |
setRotation() | Rotates a shape around the given point. |
setRotationByAnchor() | Rotates a shape around the given anchor. |
setTransformationMatrix() | Sets the transformation matrix. |
translate() | Moves a shape taking an account the current transformation. |
zIndex() | Z-index setting. |
Miscellaneous | |
attr() | Attribute setting |
clip() | Clipping setting. |
cursor() | Cursor type. |
desc() | Description setting |
disablePointerEvents() | Pointer events setting. |
disableStrokeScaling() | Vector effect property |
dispose() | Disposes element completely. |
domElement() | Returns DOM element if element is rendered. |
getStage() | Stage object (to which the given element is bound). |
hasParent() | Whether parent element is set. |
id() | Element identifier |
parent() | Parent layer. |
remove() | Current element removes itself from the parent layer. |
strokeThickness() | Stroke thickness setting. |
title() | Title setting |
Methods Description
appendTransformationMatrix
Illustration shows a shape transformation with the several calls of
.appendTransformationMatrix(0, 0.5, 1, 0, 0, 0)
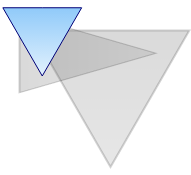
Params:
Name | Type | Description |
---|---|---|
m00 | number | Scale X. |
m10 | number | Shear Y. |
m01 | number | Shear X. |
m11 | number | Scale Y. |
m02 | number | Translate X. |
m12 | number | Translate Y. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
attr
Params:
Name | Type | Description |
---|---|---|
key | string | Name of attribute. |
Returns:
* - Attribute value.Try it:
Params:
Name | Type | Description |
---|---|---|
key | string | Name of attribute. |
value | * | Value of attribute. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
clip
Returns:
anychart.graphics.math.Rect - An instance of the class for method chaining.Attention! In SVG clip will transform according to transformation, and in VML clip will be surrounding.
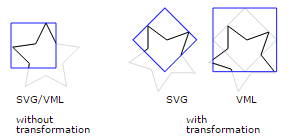
Params:
Name | Type | Description |
---|---|---|
value | anychart.graphics.math.Rect | string | Clipping rectangle. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
cursor
Returns:
anychart.graphics.vector.Cursor - Current cursor type.Try it:
Params:
Name | Type | Default | Description |
---|---|---|---|
value | anychart.graphics.vector.Cursor | null | Cursor type. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
desc
Returns:
string | null | undefined - The element description value.Try it:
Params:
Name | Type | Description |
---|---|---|
value | string | null | Value to set. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
disablePointerEvents
Returns:
boolean - Returns property value.Try it:
Params:
Name | Type | Default | Description |
---|---|---|---|
value | boolean | false | Pointer events property value. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
disableStrokeScaling
Returns:
boolean - The current vector effect property.var element = stage.rect(10, 10, 100, 40); element.disableStrokeScaling();
Learn more by link.
Params:
Name | Type | Default | Description |
---|---|---|---|
value | boolean | false | Vector effect property to set. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
dispose
Try it:
domElement
In case of Stage in Suspended state or unbound element – null is returned.
Returns:
Element - DOM element.drag
Returns:
boolean | anychart.graphics.math.Rect - Absolute element bounds or flag.Try it:
Params:
Name | Type | Default | Description |
---|---|---|---|
value | boolean | anychart.graphics.math.Rect | false | Flag or a dragging area. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
fill
Returns:
anychart.graphics.vector.Fill - The current fill (empty fill is 'none").Try it:
Params:
Name | Type | Description |
---|---|---|
value | anychart.graphics.vector.Fill | Fill as object or string. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
Params:
Name | Type | Description |
---|---|---|
color | string | Color as string. |
opacity | number | Opacity. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
- ObjectBoundingBox with angle value preservation
- ObjectBoundingBox without angle value preservation
- UserSpaceOnUse
Modes:
ObjectBoundingBox without angle value preservation (the first rectangle on the image)In this mode the result angle will visually correspond the original setting, non regarding browser scaling duplication (so, for objects that do not have 1:1 proportion with the original figure, the gradient angle will correspond to the initial value due to internal calculations).
ObjectBoundingBox with angle value preservation(the second rectangle on the image)
In this mode, gradient vector is calculated with the preset angle, but the result gradient angle on the rendered page can be changed if the object proportion is not 1:1 in the browser. So visually the result gradient angle may not correspond to the original settings.
UserSpaceOnUse (the third and fourth rectangles on the image)
In this mode gradient settings are added by gradient size and borders/coordinates, and rendering is calculated within those borders. After that, the fill is executed on the element figure according to its coordinates. More about this mode at gradientUnits.
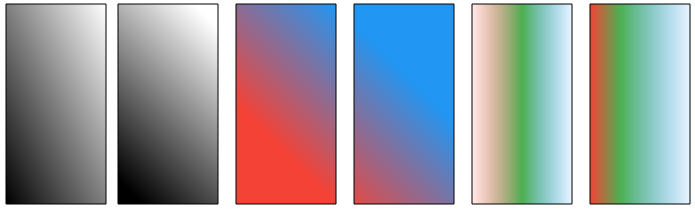
Params:
Name | Type | Description |
---|---|---|
keys | Array.<(anychart.graphics.vector.GradientKey|string)> | Gradient keys. |
angle | number | Gradient angle. |
mode | boolean | anychart.graphics.vector.Rect | Object | Gradient mode. |
opacity | number | Gradient opacity. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
Params:
Name | Type | Description |
---|---|---|
keys | Array.<(anychart.graphics.vector.GradientKey|string)> | Color-stop gradient keys. |
cx | number | X ratio of center radial gradient. |
cy | number | Y ratio of center radial gradient. |
mode | anychart.graphics.math.Rect | If defined then userSpaceOnUse mode else objectBoundingBox. |
opacity | number | Opacity of the gradient. |
fx | number | X ratio of focal point. |
fy | number | Y ratio of focal point. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
getAbsoluteBounds
Returns:
anychart.graphics.math.Rect - Absolute element bounds.Try it:
getAbsoluteHeight
See illustrations at anychart.graphics.vector.Element#getAbsoluteWidth
Returns:
number - Height.Try it:
getAbsoluteWidth
getAbsoluteX
Returns:
number - Absolute X.Try it:
getAbsoluteY
Returns:
number - Absolute Y.Try it:
getBounds
getHeight
getRotationAngle
getStage
Returns:
anychart.graphics.vector.Stage - Stage object.Try it:
getTransformationMatrix
[
{number} m00 Scale X.
{number} m10 Shear Y.
{number} m01 Shear X.
{number} m11 Scale Y.
{number} m02 Translate X.
{number} m12 Translate Y.
]
Returns:
Array.<number> - Transformation matrix array.Try it:
getWidth
getX
Returns:
number - X in the coordinate system of the parent.Try it:
getY
Returns:
number - Y in the coordinate system of the parent.Try it:
hasParent
id
Returns:
string - Returns element identifier.Try it:
Params:
Name | Type | Description |
---|---|---|
value | string | Custom identifier. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
listen
Params:
Name | Type | Default | Description |
---|---|---|---|
type | string | The event type id. | |
listener | function | Callback method. | |
useCapture | boolean | false | Whether to fire in capture phase. Learn more about capturing https://javascript.info/bubbling-and-capturing |
listenerScope | Object | Object in whose scope to call the listener. |
Returns:
Object - Unique key for the listener.Try it:
listenOnce
Params:
Name | Type | Default | Description |
---|---|---|---|
type | string | The event type id. | |
listener | function | Callback method. | |
useCapture | boolean | false | Whether to fire in capture phase. Learn more about capturing https://javascript.info/bubbling-and-capturing |
listenerScope | Object | Object in whose scope to call the listener. |
Returns:
Object - Unique key for the listener.Try it:
parent
Returns:
anychart.graphics.vector.Layer | anychart.graphics.vector.Stage - Instance of element current layer.Try it:
Params:
Name | Type | Description |
---|---|---|
value | anychart.graphics.vector.Layer | anychart.graphics.vector.Stage | Parent layer. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
remove
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
removeAllListeners
Params:
Name | Type | Description |
---|---|---|
type | string | Type of event to remove, default is to remove all types. |
Returns:
number - Number of listeners removed.Try it:
rotate
Left illustration shows 20 degrees rotation with the default rotation point.
.rotate(20)
Right illustration shows 20 degrees rotation with the given rotation point.
.rotate(20, x, y)
Rotation points are marked with red, initial position of shapes is marked with gray.
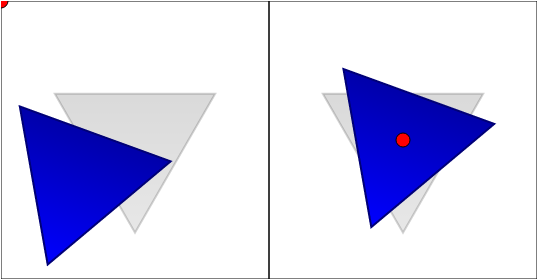
Params:
Name | Type | Description |
---|---|---|
degrees | number | Rotation angle in degrees. |
cx | number | Rotation point X. |
cy | number | Rotation point Y. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
rotateByAnchor
Left illustration shows 90 degrees rotation around the default anchor.
.rotateByAnchor(90)
Right illustration shows 90 degrees rotation around the given anchor.
.rotateByAnchor(90, anychart.graphics.vector.Anchor.CENTER_TOP)
Rotation points are marked with red, initial position of shapes is marked with gray.
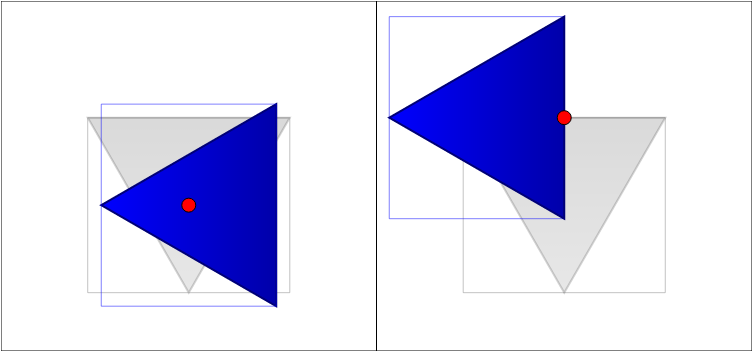
Params:
Name | Type | Description |
---|---|---|
degrees | number | Rotation angle in degrees. |
anchor | anychart.graphics.vector.Anchor | string | Rotation anchor. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
scale
Left illustration shows default point scaling.
.scale(0.5, 0.5,)
Right illustration shows scaling with the scaling center set.
.scale(0.5, 0.5, x, y)
Scaling center is marked with red, initial state is marked with gray.
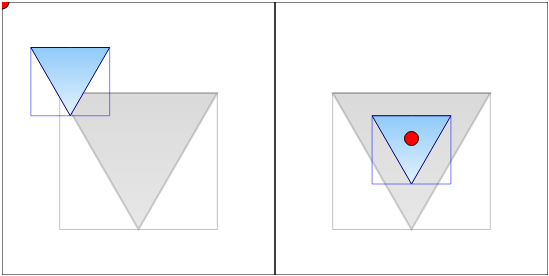
Params:
Name | Type | Description |
---|---|---|
sx | number | X scaling factor. |
sy | number | Y scaling factor. |
cx | number | Scaling point X. |
cy | number | Scaling point Y. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
scaleByAnchor
Left illustration shows scaling with the default anchor.
.scaleByAnchor(0.5, 0.5)
Right illustration shows scaling with the anchor set.
.scaleByAnchor(0.5, 0.5, anychart.graphics.vector.Anchor.RIGHT_TOP)
Scaling center is marked with red, initial state is marked with gray.
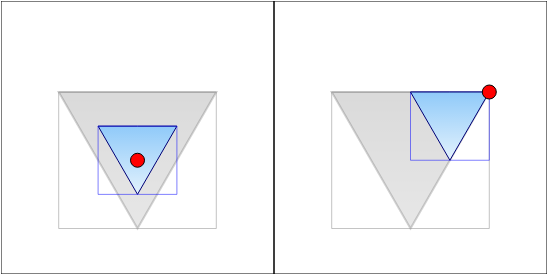
Params:
Name | Type | Description |
---|---|---|
sx | number | X scaling factor. |
sy | number | Y scaling factor. |
anchor | anychart.graphics.vector.Anchor | string | Scaling anchor point. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
setPosition
Params:
Name | Type | Description |
---|---|---|
x | number | X coordinate. |
y | number | Y coordinate. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
setRotation
Params:
Name | Type | Description |
---|---|---|
degrees | number | Rotation angle in degrees. |
cx | number | Rotation point X. |
cy | number | Rotation point Y. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
setRotationByAnchor
Params:
Name | Type | Description |
---|---|---|
degrees | number | Rotation angle in degrees. |
anchor | anychart.graphics.vector.Anchor | string | Rotation anchor. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
setTransformationMatrix
Params:
Name | Type | Description |
---|---|---|
m00 | number | Scale X. |
m10 | number | Shear Y. |
m01 | number | Shear X. |
m11 | number | Scale Y. |
m02 | number | Translate X. |
m12 | number | Translate Y. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
stroke
- As "[thickness ]color[ opacity]" formatted string:
- "color" - https://www.w3schools.com/html/html_colors.asp.
- "thickness color" - like a css border, e.g. "3 red" or "3px red"
- "color opacity" - as a fill string, e.g. "#fff 0.5"
- "thickness color opacity" - as a complex string, e.g. "3px #00ff00 0.5"
- anychart.graphics.vector.Stroke object
- Array of anychart.graphics.vector.GradientKey keys
- null resets current stroke.
Params:
Name | Type | Description |
---|---|---|
value | anychart.graphics.vector.Stroke | anychart.graphics.vector.ColoredFill | string | null | Stroke as '[thickness ]color[ opacity]'. return {anychart.graphics.vector.Shape} Self instance for method chaining. |
Params:
Name | Type | Description |
---|---|---|
value | anychart.graphics.vector.Stroke | anychart.graphics.vector.ColoredFill | string | Stroke style, as described above. |
thickness | number | Line thickness. Defaults to 1. |
dashpattern | string | Controls the pattern of dashes and gaps used to stroke paths. Dash array contains a list of white space separated lengths and percentages that specify the lengths of alternating dashes and gaps. If an odd number of values is provided, then the list of values is repeated to yield an even number of values. Thus, stroke dashpattern: "5 3 2" is equivalent to dashpattern: "5 3 2 5 3 2". |
lineJoin | anychart.graphics.vector.StrokeLineJoin | Line join style. |
lineCap | anychart.graphics.vector.StrokeLineCap | Line cap style. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
strokeThickness
Params:
Name | Type | Description |
---|---|---|
value | number | Thickness value. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
title
Returns:
string | null | undefined - The element title value.Try it:
Params:
Name | Type | Description |
---|---|---|
value | string | null | Value to set. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
translate
Illustration shows a shape movement when
.translate(20, 10)
is invoked several times.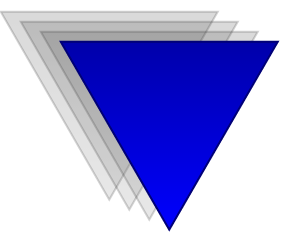
Params:
Name | Type | Description |
---|---|---|
tx | number | X movement amount. |
ty | number | Y movement amount. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
unlisten
Params:
Name | Type | Default | Description |
---|---|---|---|
type | string | The event type id. | |
listener | function | Callback method. | |
useCapture | boolean | false | Whether to fire in capture phase. Learn more about capturing https://javascript.info/bubbling-and-capturing |
listenerScope | Object | Object in whose scope to call the listener. |
Returns:
boolean - Whether any listener was removed.Try it:
visible
Returns:
boolean - Returns the current visibility flag.Try it:
Params:
Name | Type | Default | Description |
---|---|---|---|
isVisible | boolean | true | Visibility flag. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it:
zIndex
Params:
Name | Type | Description |
---|---|---|
value | number | Z-index to set. |
Returns:
anychart.graphics.vector.Shape - Self instance for method chaining.Try it: