class anychart.graphics.vector.Path Improve this Doc
Path class.
Path is sequence of segments of different type, it can be opened or closed.
To define the internal fill this rule is used EVEN-ODD.
Path always starts with anychart.graphics.vector.Path#moveTo command.
Do not invoke constructor directly. Use anychart.graphics.vector.Stage#path or
anychart.graphics.vector.Layer#path to create stage or layer bound path.
To create unbound path use anychart.graphics#path
See also:
anychart.graphics.vector.Stage#path
anychart.graphics.vector.Layer#path
anychart.graphics#path
Methods Overview
Miscellaneous | |
arcTo() | Adds a command to the path that draws an arc of an ellipse. |
arcToAsCurves() | This method is similar to anychart.graphics.vector.Path#arcTo, but in this case the arc is approximated by Bezier curves. |
arcToByEndPoint() | Adds a command to the path that draws an arc of an ellipse. |
circularArc() | Adds a command to the path that draws a circular arc. |
clear() | Resets all path operations. |
close() | Adds a command that closes the path by connecting the last point with the first straight line. |
curveTo() | Adds specified points to the path, drawing sequentially a cubic Bezier curve from the current point to the next. |
getCurrentPoint() | Returns the last coordinates added to the path. |
lineTo() | Adds specified points to the current path, drawing sequentially a straight line through the specified coordinates. |
moveTo() | Moves path cursor position to a specified coordinate. |
quadraticCurveTo() | Adds specified points to the path, drawing sequentially a quadratic Bezier curve from the current point to the next. |
Methods Description
arcTo
An ellipse with radius rx, ry, starting from an angle fromAngle, with an angular length extent.
The positive direction is considered the direction from a positive direction of the X-axis to a positive direction of the Y-axis, that is clockwise.
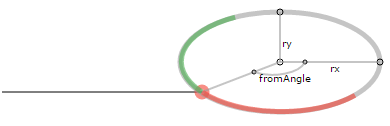
The black line marks the current path.
The red point is the point from which the arc is drawn.
According to the given parameters, an ellipse is plotted with radii rx and ry, and an angle fromAngle, which defines the position of the ellipse against the red point.
Then an ellipse arc of a given angular length extend is drawn (the arc is marked green if extend > 0 and red if extend < 0).
Params:
Name | Type | Description |
---|---|---|
rx | number | The X-axis radius of the ellipse. |
ry | number | The Y-axis radius of the ellipse. |
fromAngle | number | The starting angle, measured in degrees in a clockwise direction. |
extent | number | The angular length of the arc. |
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.Try it:
arcToAsCurves
java.awt.geom.ArcIterator algorithm adaptation
You can find an illustration of how the method works, and examples in the anychart.graphics.vector.Path#arcTo method description.
The only difference is that this method draws an arc using a set of curves.
Params:
Name | Type | Description |
---|---|---|
rx | number | The X-axis radius of the ellipse. |
ry | number | The Y-axis radius of the ellipse. |
fromAngle | number | The starting angle, measured in degrees in a clockwise direction. |
extent | number | The angular length of the arc. |
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.arcToByEndPoint
An arc of an ellipse with radius rx, ry rx, ry from the current point to a point x, y.
The largeArc and clockwiseArc flags define which of the 4 possible arcs is drawn.
Read more at https://www.w3.org/TR/SVG/implnote.html#ArcImplementationNotes
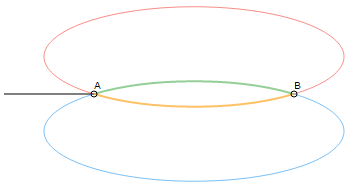
There are several ways to get from point A a to point B, given the same x, y, rx, ry parameters. The way to get to point B, is defined by the pair largeArc, clockwiseArc:
- largeArc – defines if the larger (red and blue) or the smaller (green and yellow) arc is drawn;
- clockwiseArc – defines if the arc is drawn clockwise (red and green) or counterclockwise (yellow and blue).
Params:
Name | Type | Description |
---|---|---|
x | number | The X-coordinate of the arc end. |
y | number | The Y-coordinate of the arc end. |
rx | number | The X-axis radius of the ellipse. |
ry | number | The Y-axis radius of the ellipse. |
largeArc | boolean | A flag allowing to draw either the smaller or the larger arc. |
clockwiseArc | boolean | A flag allowing to draw an arc either in a clockwise or in a counterclockwise direction. |
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.Try it:
circularArc
An arc with a center in (cx, cy) start angle (from) and end angle (from + sweep), with clockwise and counterclock drawing option.
Params:
Name | Type | Default | Description |
---|---|---|---|
cx | number | Center x. | |
cy | number | Center y. | |
rx | number | The X-axis radius of the ellipse. | |
ry | number | The Y-axis radius of the ellipse. | |
fromAngle | number | The starting angle, measured in degrees in a clockwise direction. | |
sweep | number | Sweep angle in degrees. | |
lineTo | boolean | false | Line to start point. If set to true - anychart.graphics.vector.Path#lineTo will be used instead of anychart.graphics.vector.Path#moveTo. |
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.Try it:
clear
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.Try it:
close
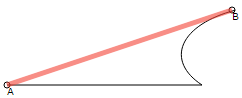
Assume that some path has been being drawn. After calling the close method, the current point B will be connected with the beginning of the path (point A) by a straight line (marked red).
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.Try it:
curveTo
Each curve is defined by 3 points (6 coordinates) – two control points and an endpoint.
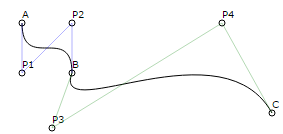
The cursor is set to the point A.
Then the curve goes to the point B through the inflection points P1 and P2.
Next the curve goes to the point C through the inflection points P3 and P4.
Inflection points can be placed anywhere, but there must be two of them.
Params:
Name | Type | Description |
---|---|---|
control1X | number | The first control point’s X-coordinate. |
control1Y | number | The first control point’s Y-coordinate. |
control2X | number | The second control point’s X-coordinate. |
control2Y | number | The second control point’s Y-coordinate. |
endX | number | The endpoint’s X-coordinate. |
endY | number | The endpoint’s Y-coordinate. |
var_args | number | The coordinates, defining curves, in sets of 6: first control points, then an endpoint (in the same order as the primary parameters). |
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.getCurrentPoint
Returns:
anychart.math.Coordinate - The current coordinates of the cursor.Try it:
lineTo
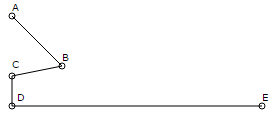
The beginning is in the point A(50, 50).
The first line is drawn from the beginning to the point B(100, 100).
Then the path goes on to the point C(50, 110).
From the point C it goes to the point D(50, 140)
and ends in the point E(300, 140).
Params:
Name | Type | Description |
---|---|---|
x | number | A target point’s X-coordinate. |
y | number | A target point’s Y-coordinate. |
var_args | number | The target points’ coordinates: each odd parameter is interpreted as X and each even as Y. |
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.moveTo
Params:
Name | Type | Description |
---|---|---|
x | number | The target point’s X-coordinate. |
y | number | The target point’s Y-coordinate. |
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.Try it:
quadraticCurveTo
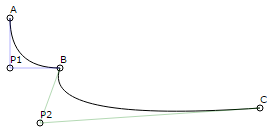
The cursor is set to the point A.
Then the curve goes to the point B through the inflection point P1.
Next the curve goes to the point C through the inflection point P2.
The inflection point can be placed anywhere, and there must be one such point.
Params:
Name | Type | Description |
---|---|---|
controlX | number | The control point’s X-coordinate. |
controlY | number | The control point’s Y-coordinate. |
endX | number | The endpoint’s X-coordinate. |
endY | number | The endpoint’s Y-coordinate. |
var_args | number | coordinates, defining curves, in sets of four: first the control point, then an endpoint (in the same order as the primary parameters). |
Returns:
anychart.graphics.vector.Path - Self instance for method chaining.